Terminal-based Text Editors (nano)¶
After learning a few commands the next thing to learn is to edit text files. As we will see later, editing small text files is a common procedure on an HPC cluster; for example, the submission scripts are relatively small text files, and most of the time, you have to make small adjustments to those scripts. Many scientific codes use text-based input files for running simulations. You need to know how to use at least one basic editor to help you with those tasks.
There are several editors available on modern UNIX/Linux machines. The most widely used are vi, emacs, and nano.
The standard de facto editor in UNIX is vi and a hacker favorite. The Single UNIX Specification (SUS) specifies vi, so every conforming system must have it.
The second most commonly used editor is emacs, sometimes not installed by default on many systems it is usually provided on most Linux distributions.
The third editor is GNU nano a very user-friendly editor. For this tutorial we will focus on nano, for short text files and simple edits, nano serves its purpose.
Opening GNU nano¶
On a terminal execute:
$> nano <name_of_file>
You will get a text application that looks like this:
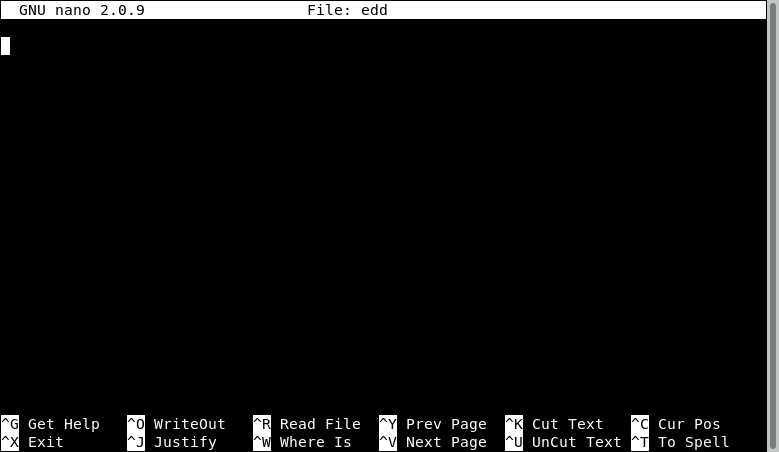
Using GNU nano¶
The bottom two lines show the commands to use.
The symbol ^
means press the CONTROL KEY (left or right) and keep it pressed until you press the command key. For example, to exit the editor we see ^X
, which tells us that we must hold the control key and then press X
. The character in the command is not case sensitive so pressing ^X
is equivalent to ^x
.
Executing commands with nano will sometimes where st of subcommands; some of them will be written with an M
(eg. M-C
), in that case M
indicates the META
character, which corresponds to Alt
on modern keyboards.
The command ^G
display a complete list of keystrokes available from the main window. To exit from the help page, execute ^X
GNU nano offers a fairly complete set of basic utilities to edit files.
Search for text with ^W
and the text to find.
The command ^_
allows you to go to a specific line number.
Copy, Cut and Paste¶
The command ^K
and ^U
only serve to cut and paste the line where the cursor is located. When you cut the text, its contents are stored in what is called the cut buffer. The command ^U
just takes the contents of the cut buffer and write its contents to the location of the cursor. As the contents of the cut buffer are not erased you can use ^U
to repeat the same contents as many times as you want.
For more advanced copy and paste, you need to learn how to select blocks of text before cutting them to the cut buffer
First, move the cursor to the start of the text you want to select, press the M-A
key combination (Alt-A in modern keyboards) to mark the start, then move the cursor to the end of the section you want to select.
Once you have marked the beginning and end of the text, the ^^
(Esc-6) and ^K
key combinations can be used to copy or cut it, respectively.
In a command like ^^
, the meaning of that is to press the Control Key followed by the carret symbol ^
.
Quitting Nano¶
To quit nano, use the ^X
key combination. If the file you are working on has been modified since the last time you saved it, you will be asked to save the file first. Type y to save the file, or n to exit nano without saving the file.
Exercise¶
As an exercise, take the opportunity to write the source code of a simple parallel program, open nano and write the code below:
#include <mpi.h>
#include <stdio.h>
int main(int argc, char** argv) {
// Initialize the MPI environment
MPI_Init(NULL, NULL);
// Get the number of processes
int world_size;
MPI_Comm_size(MPI_COMM_WORLD, &world_size);
// Get the rank of the process
int world_rank;
MPI_Comm_rank(MPI_COMM_WORLD, &world_rank);
// Get the name of the processor
char processor_name[MPI_MAX_PROCESSOR_NAME];
int name_len;
MPI_Get_processor_name(processor_name, &name_len);
// Print off a hello world message
printf("Hello world from processor %s, rank %d out of %d processors\n",
processor_name, world_rank, world_size);
// Finalize the MPI environment.
MPI_Finalize();
}
The code is in C, a well-known programming language for a variety of purposes, including scientific computing. In C, tabulations are not mandatory but help the legibility of the code, pay special attention to semicolons at the end of each instruction. All lines starting with a double slash //
are comments, so you can ignore those lines if you want.
In the next chapter, we will show how to compile the code and execute it on the cluster using the queue manager.